To fix the error “dirname is not defined in ES module scope,” you can use the import.meta.url method, create a wrapper function, or use a configuration file with conditional logic for mixed environments. Another option is to use the console.log(__dirname) method in Node with ES Modules or install the splendid-ui package and import dirname from ‘splendid-ui/node’.
It’s important to note that __dirname is a local variable that returns the directory name of the current module or the folder path of the current JavaScript file. This error often occurs when migrating from CommonJS to ES modules.
Table of Contents
ToggleIntroduction To Es Modules And __dirname
When using ES modules, you may encounter the error message “__dirname is not defined in ES module scope”. To fix this, you can use methods such as importing import. meta. url, creating a wrapper function, or using a configuration file.
These methods allow you to obtain the directory name in ES modules.
The Shift From Commonjs To Es Modules
ES Modules or ECMAScript modules are a standardized format of organizing code. It provides a way for developers to create modular code that can be shared across multiple files and even projects. This makes it easier to manage and maintain large codebases. With the increasing popularity of ES Modules, many developers are shifting from CommonJS to ES Modules.Why __dirname Is Missing In Es Modules
One major difference between CommonJS and ES Modules is the way they handle module scope. ES Modules have a different scope than CommonJS modules, which means that some variables and objects that were available in CommonJS may not be available in ES Modules. One such variable is __dirname. In CommonJS, __dirname is a global variable that provides the directory path of the current module. However, in ES Modules, __dirname is not defined in the module scope. This means that developers cannot use __dirname to get the directory path of the current module in ES Modules.How To Fix __dirname Is Not Defined In Es Module Scope
If you encounter the error “__dirname is not defined in ES module scope”, there are several ways to fix it:- Using import.meta.url: One way to get the directory name in ES Modules is by using the import.meta.url property. This property contains the URL of the current module and can be used to derive the directory path.
- Using a Wrapper Function: We can create a wrapper function to provide the __dirname and __filename values in ES Modules. This involves passing the __dirname and __filename values as arguments to the wrapper function.
- Using a Configuration File: Another way to fix the __dirname is not defined error is by using a configuration file. This involves creating a configuration file that exports the __dirname value.
- Conditional Logic for Mixed Environments: If you are working with both CommonJS and ES Modules in the same project, you can use conditional logic to handle the __dirname value based on the module type.
Credit: github.com
Common Pitfalls When Migrating To Es Modules
When migrating to ES Modules, developers often encounter Common Pitfalls that can hinder the process. Among these challenges, Errors encountered during migration are a significant obstacle. One such error that developers frequently face is the __dirname is Not Defined in ES Module Scope. Understanding this error and its resolution is crucial for a smooth migration process.
Errors Encountered During Migration
During the migration to ES Modules, developers may encounter various errors that can impede the process. One common issue is the __dirname is Not Defined error, which arises due to the differences between CommonJS and ES Modules. Resolving such errors is essential for a successful migration to ES Modules.
Understanding The __dirname Error
The __dirname is Not Defined error occurs when attempting to access the __dirname variable in an ES Module. Unlike in CommonJS, where __dirname is readily available, ES Modules do not inherently support this variable. As a result, developers must implement alternative approaches to achieve the same functionality in ES Modules.
Using Import.meta.url To Emulate __dirname
When working with ES modules, the absence of __dirname can pose a challenge for developers. However, by utilizing the import.meta.url feature, it is possible to emulate the functionality of __dirname in ES module scope. This allows for obtaining the directory path in a modular, ES6-compliant manner.
Steps To Use Import.meta.url
- Access the URL using import.meta.url
- Convert the URL to a directory path
Converting Url To Directory Path
To convert the obtained URL to a directory path, developers can use the following code snippet:
const path = new URL('.', import.meta.url).pathname;
const directoryPath = decodeURIComponent(path);
Creating A Wrapper Function For __dirname
To resolve the issue of “__dirname is not defined in ES module scope,” you can create a wrapper function to provide the __dirname and __filename values in the ES modules. This can be achieved by using import. meta. url or a configuration file, or by implementing conditional logic for mixed environments.
Designing The Wrapper Function
When working with ES modules in Node.js, you may come across the issue of “__dirname is not defined in ES module scope”. This error occurs because the __dirname variable is not available in the ES module scope like it is in CommonJS modules.
To resolve this issue, one approach is to create a wrapper function that provides the __dirname and __filename values in the ES modules. This wrapper function can be designed to mimic the behavior of the __dirname variable in CommonJS modules.
Implementing __dirname And __filename
To implement the wrapper function, you can use the import.meta.url property, which provides the URL of the current module. From this URL, you can extract the directory path using the path.dirname() function.
Here’s an example of how the wrapper function can be implemented:
import { fileURLToPath } from 'url';
import { dirname } from 'path';
function getDirname() {
const __filename = fileURLToPath(import.meta.url);
const __dirname = dirname(__filename);
return __dirname;
}
In this example, the fileURLToPath() function is used to convert the import.meta.url to a file path. The dirname() function then extracts the directory name from the file path.
By calling the getDirname() function, you can now obtain the directory name in the ES modules, similar to how __dirname works in CommonJS modules.
With the wrapper function in place, you can use it throughout your ES modules to access the directory name. This allows you to maintain compatibility with existing code that relies on __dirname.
By creating a wrapper function for __dirname in ES module scope, you can overcome the error of “__dirname is not defined”. This solution provides a way to access the directory name in ES modules, allowing for seamless migration from CommonJS modules to ES modules.
Leveraging Configuration Files
To fix the error “__dirname is not defined in ES module scope,” there are a few approaches you can take. One option is to use the import. meta. url method to get the directory name in ES modules. Another approach is to create a wrapper function that provides the __dirname and __filename values.
Additionally, you can use a configuration file or implement conditional logic for mixed environments. These methods will help resolve the issue and allow you to leverage configuration files effectively.
Setting Up Configuration For Directory Names
When working with ES modules in Node.js, the `__dirname` variable is not defined in the module scope. This can be problematic when you need to reference the current working directory or the directory of the current module. One solution is to set up a configuration file that provides the necessary directory information. You can create a `config.js` file and define the `__dirname` variable there. For example: “`javascript // config.js export const __dirname = new URL(‘.’, import.meta.url).pathname; “` Then, in your module files, you can simply import the `__dirname` variable from the configuration file: “`javascript // index.js import { __dirname } from ‘./config.js’; console.log(__dirname); // prints the directory of the current module “`Automating __dirname Setup
Setting up a configuration file for every module can be tedious, especially in large projects. To automate the process, you can use a build tool like webpack or Rollup. For example, with webpack, you can use the `DefinePlugin` to define the `__dirname` variable for all modules: “`javascript // webpack.config.js const path = require(‘path’); const webpack = require(‘webpack’); module.exports = { // … plugins: [ new webpack.DefinePlugin({ __dirname: JSON.stringify(path.resolve(__dirname)), }), ], }; “` Now, you can use `__dirname` in your modules without any issues: “`javascript // index.js console.log(__dirname); // prints the directory of the current module “` With this setup, you can ensure that all your modules have access to the `__dirname` variable without having to define it manually every time. Overall, leveraging configuration files is an effective way to handle the `__dirname` variable in ES module scope. By setting up a configuration file or automating the process with a build tool, you can avoid the common error of `__dirname is not defined in ES module scope` and ensure that your code runs smoothly.Conditional Logic For Mixed Environments
To fix the error “__dirname is not defined in ES module scope”, you can use conditional logic for mixed environments. One way is to use import. meta. url to get the directory name in ES modules. Another option is to create a wrapper function to provide the __dirname value.
Additionally, you can use a configuration file to handle this issue. These solutions will help you resolve the problem and ensure smooth functioning of your code.
Detecting Module Types
When working with mixed environments, it is important to detect the module types being used in the project. This can be done by checking the file extensions of the modules. For example, .mjs files are ES modules while .js files are CommonJS modules.Applying Conditional Fixes
Once the module types have been detected, conditional fixes can be applied to ensure that the code works in both environments. One common fix for the “__dirname is not defined in ES module scope” error is to use the “import.meta.url” property to get the current file path. Here is an example of how to use the “import.meta.url” property to get the directory name in ES modules:const __dirname = new URL('.', import.meta.url).pathname;
Another fix is to create a wrapper function that provides the “__dirname” and “__filename” values in ES modules.
Ensuring Each Heading Adheres To Html Syntax
Tags, As Shown In The Examples Above. It Is Also Important To Avoid Using Any Special Characters Or Symbols In The Heading, As This Can Break The Html Syntax. in Addition, It Is Important To Use Proper Capitalization And Spacing In The Heading To Make It More Readable And Visually Appealing. For Example, “detecting Module Types” Is More Visually Appealing And Easier To Read Than “detecting Module Types”. in Conclusion, Using Conditional Logic For Mixed Environments Can Help Ensure That Your Code Works Seamlessly In Different Environments. By Detecting Module Types And Applying Conditional Fixes, You Can Avoid Errors Like “__dirname Is Not Defined In Es Module Scope” And Ensure That Your Code Runs Smoothly In All Environments.
Using Third-party Libraries
When it comes to working with directory paths in ES modules, one common issue that developers may encounter is the error message “__dirname is not defined in ES module scope.” This error occurs because the __dirname variable, which is commonly used in CommonJS modules, is not available in the ES module scope.
Popular Libraries For Directory Paths
To overcome this limitation and effectively work with directory paths in ES modules, developers can utilize various third-party libraries. These libraries provide alternative solutions and make it easier to handle directory-related operations. Here are a few popular libraries:
- Path: The Path module is a built-in module in Node.js that provides utilities for working with file paths. It offers functions like
path.dirname()
andpath.resolve()
that can be used to manipulate directory paths. - Import-Fresh: Import-Fresh is a library that allows you to import modules and reload them every time they are required. It can be useful when dealing with dynamically changing directory paths.
- Find-Up: Find-Up is a library that searches for a file or directory by traversing parent directories. It provides a convenient way to locate files or directories relative to the current module.
Integrating Third-party Solutions
To integrate these third-party solutions into your project, you can follow these steps:
- Install the desired library using a package manager like npm or yarn. For example, to install the Path module, you can run the command
npm install path
. - Import the library in your ES module file using the
import
statement. For example, to import the Path module, you can useimport path from 'path';
. - Utilize the functions and methods provided by the library to handle directory paths in your code. For example, you can use
path.dirname()
to get the directory name of a file path.
By leveraging these third-party libraries, you can overcome the limitations of the __dirname variable in ES modules and efficiently work with directory paths in your JavaScript projects.
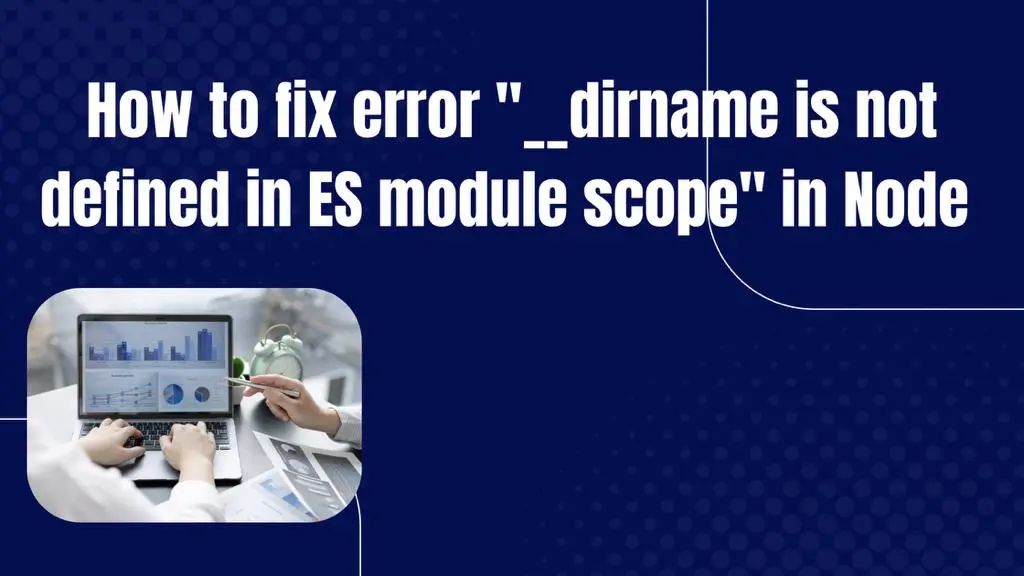
Credit: codebeautify.org
Best Practices For Es Module Development
To fix the error “__dirname is not defined in ES module scope,” there are several best practices you can follow. One way is to use the import. meta. url method to get the directory name in ES modules. Another approach is to create a wrapper function that provides the values of __dirname and __filename in the ES modules.
Additionally, you can use a configuration file or conditional logic for mixed environments. These solutions will help resolve the issue and ensure smooth ES module development.
Avoiding Common __dirname Issues
When developing ES modules, it’s important to be mindful of common issues that may arise, such as the __dirname not being defined within the module scope. This can lead to errors and unexpected behavior in your code. To avoid this, consider using alternative methods to retrieve the directory name or refactor your code to eliminate the reliance on __dirname.
Staying Future-proof With Es Modules
As the JavaScript ecosystem continues to evolve, it’s crucial to stay future-proof when developing ES modules. This means embracing best practices and utilizing features that are supported in the latest specifications. By staying informed about the latest developments and leveraging tools that promote compatibility with ES modules, you can ensure that your codebase remains resilient and adaptable to future changes in the language.
Troubleshooting And Debugging
When encountering the “__dirname is Not Defined in ES Module Scope” error in your JavaScript code, it’s essential to have a systematic approach to troubleshooting and debugging the issue. By following common troubleshooting steps and leveraging community resources for support, you can effectively address this error and ensure the smooth functioning of your code.
Common Troubleshooting Steps
When faced with the “__dirname is Not Defined in ES Module Scope” error, consider the following troubleshooting steps:
- Review the code for any misspelled variable names or syntax errors.
- Check for any missing or incorrect import statements that could be causing the issue.
- Ensure that the file structure and directory paths are accurately referenced within the code.
- Verify that the JavaScript file is being loaded as an ES module and not as a CommonJS module.
Community Resources For Support
Seeking support from the developer community can provide valuable insights and solutions when dealing with the “__dirname is Not Defined in ES Module Scope” error. Consider utilizing the following community resources:
- Online forums such as Stack Overflow and Reddit where developers actively discuss and troubleshoot similar issues.
- Developer communities on platforms like GitHub and Discord, where you can engage with peers and seek assistance.
- Official documentation and forums for the JavaScript framework or library you are working with, as they often contain troubleshooting guides and FAQs.

Credit: iamwebwiz.medium.com
Frequently Asked Questions
How To Fix __ Dirname Is Not Defined In Es Module Scope?
To fix the error “__dirname is not defined in ES module scope,” you have a few options: 1. Use import. meta. url: Import the module and use import. meta. url to get the directory name. 2. Create a wrapper function: Create a function that provides the values of __dirname and __filename in ES modules.
3. Use a configuration file: Configure your project to define __dirname in the ES module scope. 4. Implement conditional logic: Use conditional logic to handle different environments and define __dirname accordingly. These solutions will help resolve the issue and allow you to access __dirname in ES modules.
How To Get __ Dirname In Es Module?
To get __dirname in ES module, use import. meta. url in Node. js. Alternatively, create a wrapper function or a configuration file. You can also employ conditional logic for mixed environments. These methods enable access to the directory name within ES modules.
How To Define Dirname?
To define dirname in ES modules, there are a few ways. You can use import. meta. url to get the directory name, create a wrapper function to provide the __dirname and __filename values, use a configuration file, or use conditional logic for mixed environments.
__dirname is a local variable that returns the directory name of the current module in Node. js. It returns the folder path of the current JavaScript file.
What Is __ Dirname In Node Js 18?
To fix the “___dirname is Not Defined in ES Module Scope” error in Node. js 18, you can use import. meta. url or create a wrapper function. Another option is to use a configuration file or employ conditional logic for mixed environments.
These methods help resolve the issue effectively.
Conclusion
To fix the error of “__dirname is not defined in ES module scope,” there are several approaches you can take. One way is to use the import. meta. url to get the directory name in ES modules. Another option is to create a wrapper function to provide the __dirname and __filename values.
You can also use a configuration file or conditional logic for mixed environments. Additionally, you can utilize the console. log(__dirname) method to obtain the directory name in Node with ES modules. Remember to install the necessary packages or libraries to support these solutions.
By implementing these fixes, you can resolve the “__dirname is not defined” error and ensure the smooth functioning of your code.